Header File
stdarg.h - handle variable argument list
Synopsis
#include <stdarg.h>
void va_start(va_list ap, argN);
void va_copy(va_list dest, va_list src);
type va_arg(va_list ap, type);
void va_end(va_list ap);
Example
#include <stdio.h>
#include <stdarg.h>
float avg( int Count, ... )
{
va_list Numbers;
va_start(Numbers, Count);
int i,Sum = 0;
for(i = 0; i < Count; ++i )
Sum += va_arg(Numbers, int);
va_end(Numbers);
return (Sum/Count);
}
int main()
{
printf("Average of whole numbers1 : %f\n", avg(10, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9));
printf("Average of whole numbers2 : %f\n", avg(3, 10,20,30));
printf("Average of whole numbers3 : %f\n", avg(2,100,200));
printf("Average of whole numbers4 : %f\n", avg(1,300));
return 0;
}
Output:
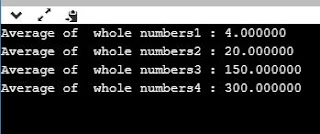
#include <stdarg.h>
void va_start(va_list ap, argN);
void va_copy(va_list dest, va_list src);
type va_arg(va_list ap, type);
void va_end(va_list ap);
Example
#include <stdio.h>
#include <stdarg.h>
float avg( int Count, ... )
{
va_list Numbers;
va_start(Numbers, Count);
int i,Sum = 0;
for(i = 0; i < Count; ++i )
Sum += va_arg(Numbers, int);
va_end(Numbers);
return (Sum/Count);
}
int main()
{
printf("Average of whole numbers1 : %f\n", avg(10, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9));
printf("Average of whole numbers2 : %f\n", avg(3, 10,20,30));
printf("Average of whole numbers3 : %f\n", avg(2,100,200));
printf("Average of whole numbers4 : %f\n", avg(1,300));
return 0;
}
Output:
No comments:
Post a Comment